Create a python program
#!/usr/bin/env python3
import subprocess
import time
import os
print(os.getpid())
p = subprocess.Popen(['/bin/sleep', '1'])
time.sleep(1000000)
In this program, the main process will start a sleep subprocess, and the execution of this sleep will end in 1 second.
At this time, it will enter the state of waiting to be recycled
However, the main process enters sleep (1000000) at this time. During the blocking process, the child process is not recycled, so the child process is not recycled
6701 main process and 6702 subprocess become zombies
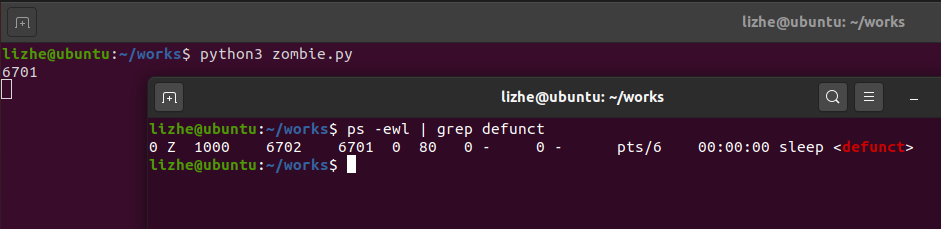
In other words, you can easily get a zombie process as long as you meet two conditions
- Parent process blocking (sleep)
- The subprocess ends execution and waits for recycling
Based on the above two conditions, let’s try docker
- Let’s first create a sleep Ubuntu container. Process 1 of this container is a blocking process, that is, it will not recycle any child processes
- We created several bash processes using bash
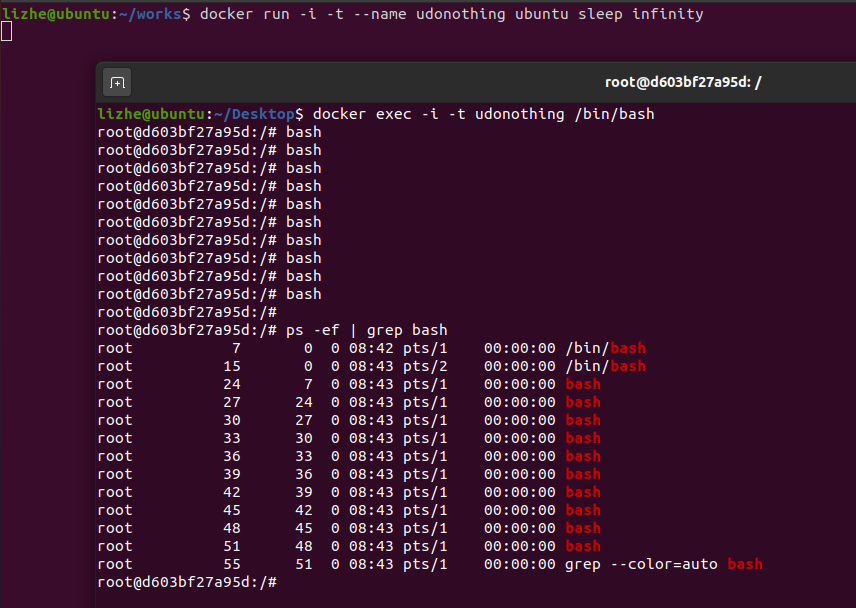
Next, let’s kill process 27, so that the parent process of process 30 will become process 1 (that is, sleep process)
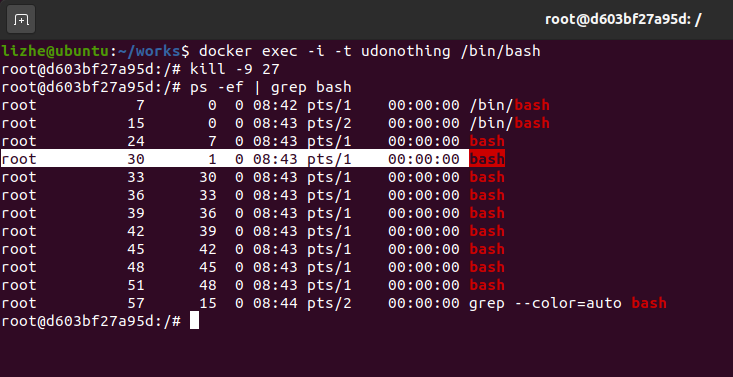
At this point, the parent process of process 30 becomes a sleep process that does not care about it
Because process 27 has been recycled by process 24, there are no zombie processes in the host
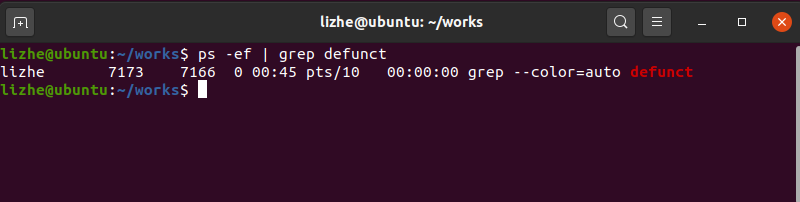
We’ll kill process 30, which nobody cares about
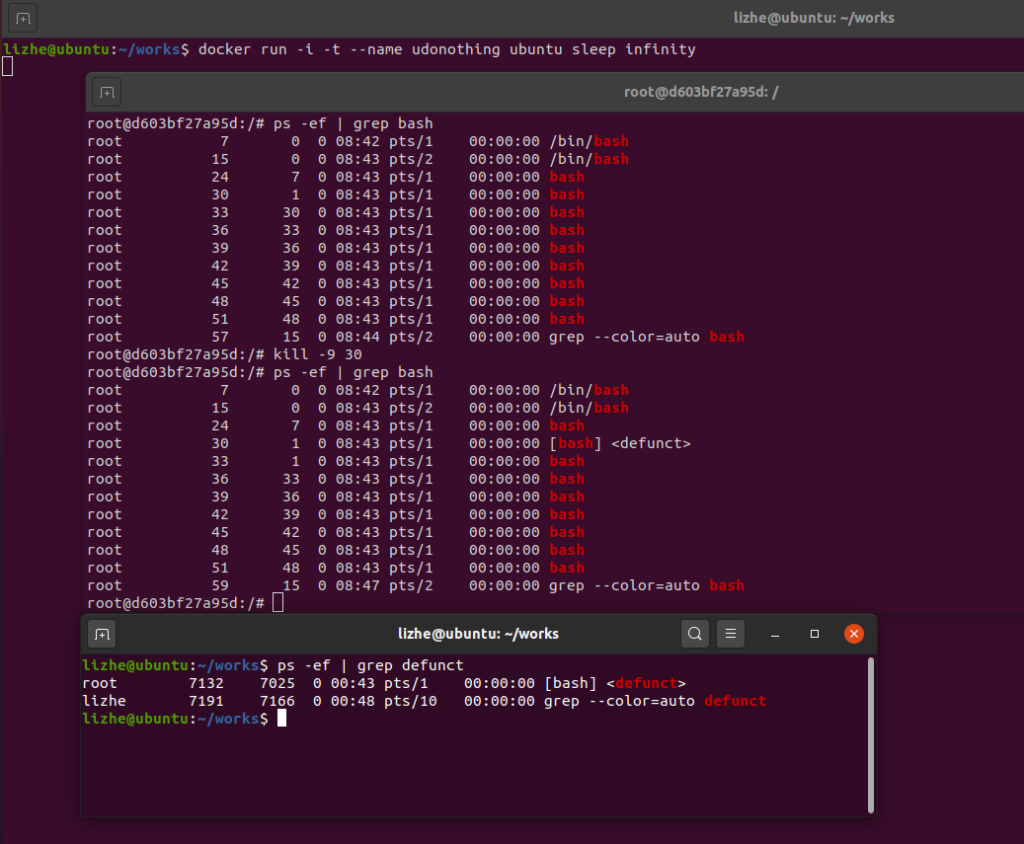
In this way, we successfully created a zombie process
PID 30 in container, PID 7132 on host
If the container is stopped, the zombie process will be recycled by the host
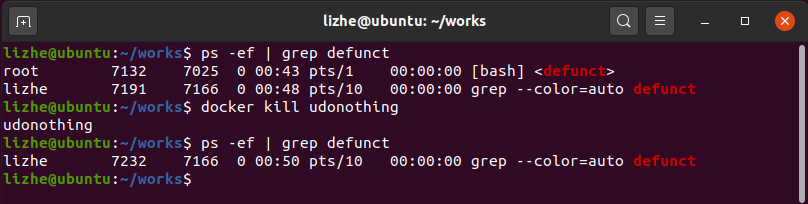
To sum up, when
- The main process of docker container is blocked for some reason
- The main process creates some child processes
- Once the parent process of these child processes becomes the container main process in blocking, they will become zombie processes that cannot be recycled after running